カバレッジと単体テスト(JUnit)を行うサンプルです。このサンプルを用いて後にJenkins連携確認を行っています。
※Jenkins連携確認がメインですので、説明はほとんど記載していません。
Eclipseのプロジェクト構成
以下のような構成でMavenプロジェクトを作成しています。
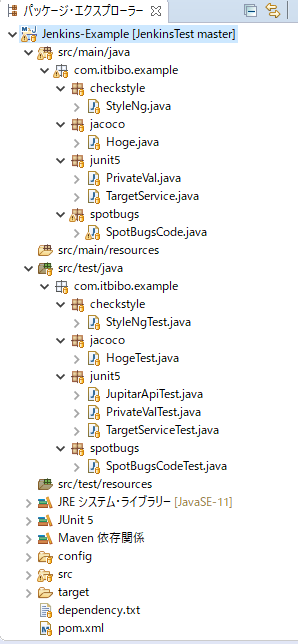
pom.xml
使用しているpom.xml、および動作確認環境は、こちらの記事のものとなります。
ソースコード
Hoge.java
package com.itbibo.example.jacoco;
public class Hoge {
/**
* sumTest.
* @param i val1
* @param j val2
* @return val
*/
public int sum(String i, String j) {
if (i == null || j == null) {
return 0;
}
return Integer.parseInt(i) + Integer.parseInt(j);
}
}
TargetService.java
package com.itbibo.example.junit5;
import java.util.ArrayList;
import java.util.List;
public class TargetService {
/**
* getItems.
* @param eq value
* @return List
*/
public List getItems(long eq) {
List retList = new ArrayList();
retList.add(String.valueOf(eq));
return retList;
}
}
PrivateVal.java
package com.itbibo.example.junit5;
/**
* privateメンバ変数テスト.
* @author itbibo
*
*/
public class PrivateVal {
@SuppressWarnings("unused")
private int testValue;
/**
* コンストラクタ.
*/
public PrivateVal() {
testValue = 0;
}
/**
* コンストラクタ.
* @param value 値
*/
public PrivateVal(int value) {
testValue = value * 2;
}
}
JUnit用テストコード
HogeTest.java
package com.itbibo.example.jacoco;
import static org.assertj.core.api.Assertions.assertThat;
import org.junit.jupiter.api.Test;
public class HogeTest {
@Test
public void addTest() {
Hoge hoge = new Hoge();
assertThat(hoge.sum("1", "2")).isEqualTo(3);
}
@Test
public void nullTest1() {
Hoge hoge = new Hoge();
assertThat(hoge.sum("1", null)).isEqualTo(0);
}
@Test
public void nullTest2() {
Hoge hoge = new Hoge();
assertThat(hoge.sum(null, "2")).isEqualTo(0);
}
}
TargetServiceTest.java
package com.itbibo.example.junit5;
import static org.assertj.core.api.Assertions.assertThat;
import static org.mockito.ArgumentMatchers.eq;
import static org.mockito.Mockito.verify;
import static org.mockito.Mockito.when;
import java.time.LocalDateTime;
import java.util.List;
import org.junit.jupiter.api.AfterEach;
import org.junit.jupiter.api.BeforeEach;
import org.junit.jupiter.api.DisplayName;
import org.junit.jupiter.api.Nested;
import org.junit.jupiter.api.RepeatedTest;
import org.junit.jupiter.api.RepetitionInfo;
import org.junit.jupiter.api.Test;
import org.junit.jupiter.api.TestInfo;
import org.junit.jupiter.api.TestReporter;
import org.junit.jupiter.api.extension.ExtendWith;
import org.junit.jupiter.params.ParameterizedTest;
import org.junit.jupiter.params.provider.CsvSource;
import org.mockito.Answers;
import org.mockito.Mock;
import org.mockito.junit.jupiter.MockitoExtension;
// MockitoExtension
@ExtendWith(MockitoExtension.class)
@DisplayName("JUnit5Example")
public class TargetServiceTest {
@Mock(answer = Answers.RETURNS_SMART_NULLS)
TargetService userService;
@BeforeEach
void setup(TestInfo testInfo) {
System.out.println("setup: " + testInfo.getDisplayName());
}
@AfterEach
void tearDown() {
System.out.println("AfterEach");
}
@Test
void userItems() {
when(userService.getItems(eq(100L))).thenReturn(List.of("Apple", "Banana", "Cherry"));
List items = userService.getItems(100L);
assertThat(items).hasSize(3);
List itemsV= verify(userService).getItems(eq(100L));
System.out.println("itemsV:" + itemsV);
}
@Test
void covTest1() {
userService = new TargetService();
List items = userService.getItems(1000);
System.out.println("items:" + items);
}
@Test
@DisplayName("TestReport")
void repoTest(TestReporter reporter) {
reporter.publishEntry("repoTest");
reporter.publishEntry("repoTest key", "repoTest value");
}
@Nested
@DisplayName("TestHoge")
static class HogeTests {
@RepeatedTest(value = 3, name = RepeatedTest.LONG_DISPLAY_NAME)
@DisplayName("hogehoge")
void hoge(RepetitionInfo info) {
System.out.println("hoge current:" + info.getCurrentRepetition()
+ " total:" + info.getTotalRepetitions());
}
}
@Nested
@DisplayName("TestFuga")
static class FugaTests {
@ParameterizedTest
@CsvSource({ "foo, 1000, 100, 2020-10-20T00:00:00.000",
"bar, 2000, 200, 2020-11-22T12:00:00.000",
"baz, 3000, 300, 2020-12-30T23:00:00.000" })
@DisplayName("fugafuga")
void fuga(String first, int second, long third, LocalDateTime fourth) {
System.out.println(
"fuga first:" + first + " second:" + second
+ " third:" + third + " fourth:" + fourth.toString());
}
}
}
PrivateValTest.java
package com.itbibo.example.junit5;
import static org.junit.jupiter.api.Assertions.*;
import java.lang.reflect.Field;
import org.junit.jupiter.api.BeforeEach;
import org.junit.jupiter.api.Test;
public class PrivateValTest {
private PrivateVal mTest1;
private PrivateVal mTest2;
public PrivateValTest() {
}
@BeforeEach
void setUp() throws Exception {
mTest1 = new PrivateVal();
mTest2 = new PrivateVal(50);
}
/**
* コンストラクタ(引数なし)のテスト
* @throws Exception
*/
@Test
public void testPrivateTestProject1() throws Exception {
//クラスオブジェクト取得
Class extends PrivateVal> c = mTest1.getClass();
//メンバ変数取得
Field fld = c.getDeclaredField("testValue");
//アクセス権限付与
fld.setAccessible(true);
assertEquals(0, ((Integer)fld.get(mTest1)).intValue());
}
/**
* コンストラクタ(引数あり)のテスト
*/
@Test
public void testPrivateTestProject2() throws Exception{
//クラスオブジェクト取得
Class extends PrivateVal> c = mTest2.getClass();
//メンバ変数取得
Field fld = c.getDeclaredField("testValue");
//アクセス権付与
fld.setAccessible(true);
assertEquals(100, ((Integer)fld.get(mTest2)).intValue());
}
}
JupitarApiTest.java
package com.itbibo.example.junit5;
import static org.assertj.core.api.Assertions.assertThat;
import static org.junit.jupiter.api.Assertions.fail;
import static org.junit.jupiter.api.Assertions.assertEquals;
import static org.junit.jupiter.api.condition.JRE.JAVA_11;
import static org.junit.jupiter.api.condition.JRE.JAVA_8;
import static org.junit.jupiter.api.condition.JRE.JAVA_9;
import static org.junit.jupiter.api.condition.OS.LINUX;
import static org.junit.jupiter.api.condition.OS.MAC;
import static org.junit.jupiter.api.condition.OS.WINDOWS;
import org.junit.jupiter.api.AfterAll;
import org.junit.jupiter.api.AfterEach;
import org.junit.jupiter.api.BeforeAll;
import org.junit.jupiter.api.BeforeEach;
import org.junit.jupiter.api.Disabled;
import org.junit.jupiter.api.DisplayName;
import org.junit.jupiter.api.Tag;
import org.junit.jupiter.api.Test;
import org.junit.jupiter.api.condition.DisabledForJreRange;
import org.junit.jupiter.api.condition.DisabledIfEnvironmentVariable;
import org.junit.jupiter.api.condition.DisabledIfSystemProperty;
import org.junit.jupiter.api.condition.DisabledOnJre;
import org.junit.jupiter.api.condition.EnabledForJreRange;
import org.junit.jupiter.api.condition.EnabledIfEnvironmentVariable;
import org.junit.jupiter.api.condition.EnabledIfSystemProperty;
import org.junit.jupiter.api.condition.EnabledOnJre;
import org.junit.jupiter.api.condition.EnabledOnOs;
@Tag("base")
public class JupitarApiTest {
JupitarApiTest() {
}
@Test
void testApp1() {
assertEquals(2, 1 + 1);
}
@Test
void testApp2() {
assertEquals(3, 2 + 2);
}
@BeforeAll
static void beforeAll() throws Exception {
System.out.println("beforeAll");
}
@AfterAll
static void afterAll() throws Exception {
System.out.println("afterAll");
}
@BeforeEach
void before() throws Exception {
System.out.println("BeforeEach before");
}
@AfterEach
void after() throws Exception {
System.out.println("AfterEach after");
}
@Test
@DisplayName("4 * 3 = 12")
void test() throws Exception {
assertThat(4 * 3).isEqualTo(12);
}
@Disabled
@Test
@DisplayName("無効")
void disabledTest() throws Exception {
fail("実行されない");
}
@Test
@EnabledOnOs(MAC)
void onlyOnMacOs() {
System.out.println("onlyOnMacOs");
}
@Test
@EnabledOnOs({ LINUX, MAC })
void onLinuxOrMac() {
System.out.println("onLinuxOrMac");
}
@Test
@EnabledOnOs(WINDOWS)
void enabledOnWindows() throws Exception {
System.out.println("enabledOnWindows");
}
@Test
@EnabledOnJre(JAVA_8)
void onlyOnJava8() {
System.out.println("onlyOnJava8");
}
@Test
@EnabledOnJre({ JAVA_8, JAVA_11 })
void onJava8Or11() {
System.out.println("onJava8Or11");
}
@Test
@EnabledForJreRange(min = JAVA_9, max = JAVA_11)
void fromJava9to11() {
System.out.println("fromJava9to11");
}
@Test
@EnabledForJreRange(min = JAVA_9)
void fromJava9toCurrentJavaFeatureNumber() {
System.out.println("fromJava9toCurrentJavaFeatureNumber");
}
@Test
@EnabledForJreRange(max = JAVA_11)
void fromJava8To11() {
System.out.println("fromJava8To11");
}
@Test
@DisabledOnJre(JAVA_9)
void notOnJava9() {
System.out.println("notOnJava9");
}
@Test
@DisabledForJreRange(min = JAVA_9, max = JAVA_11)
void notFromJava9to11() {
System.out.println("notFromJava9to11");
}
@Test
@DisabledForJreRange(min = JAVA_9)
void notFromJava9toCurrentJavaFeatureNumber() {
System.out.println("notFromJava9to11");
}
@Test
@DisabledForJreRange(max = JAVA_11)
void notFromJava8to11() {
System.out.println("notFromJava8to11");
}
@Test
@EnabledIfSystemProperty(named = "os.arch", matches = ".*64.*")
void onlyOn64BitArchitectures() {
System.out.println("onlyOn64BitArchitectures");
}
@Test
@DisabledIfSystemProperty(named = "ci-server", matches = "true")
void notOnCiServer() {
System.out.println("notOnCiServer");
}
@Test
@EnabledIfEnvironmentVariable(named = "ENV", matches = "staging-server")
void onlyOnStagingServer() {
System.out.println("onlyOnStagingServer");
}
@Test
@DisabledIfEnvironmentVariable(named = "ENV", matches = ".*development.*")
void notOnDeveloperWorkstation() {
System.out.println("notOnDeveloperWorkstation");
}
}
カバレッジ
カバレッジの取得手順です。
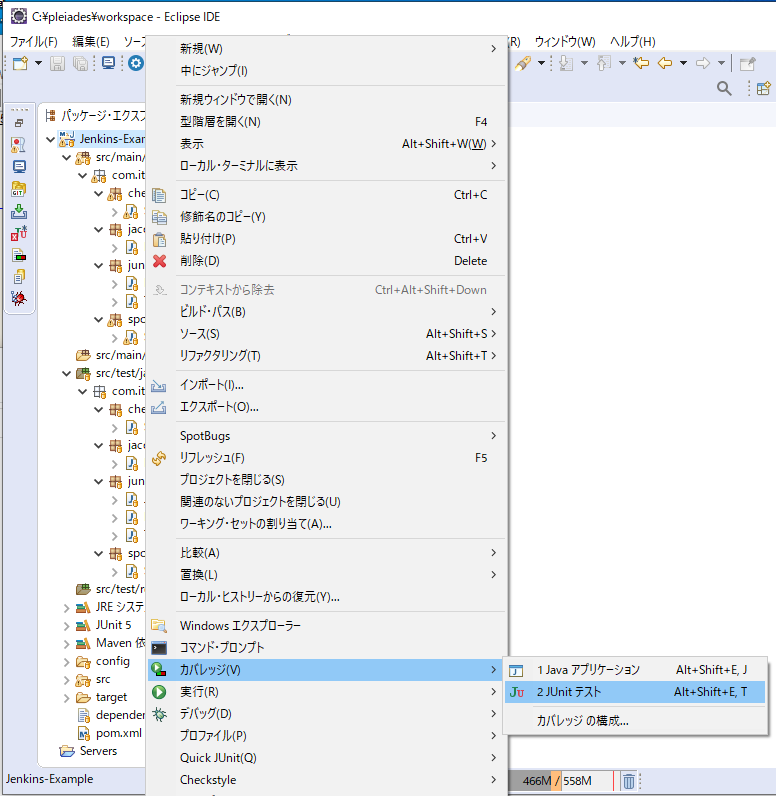
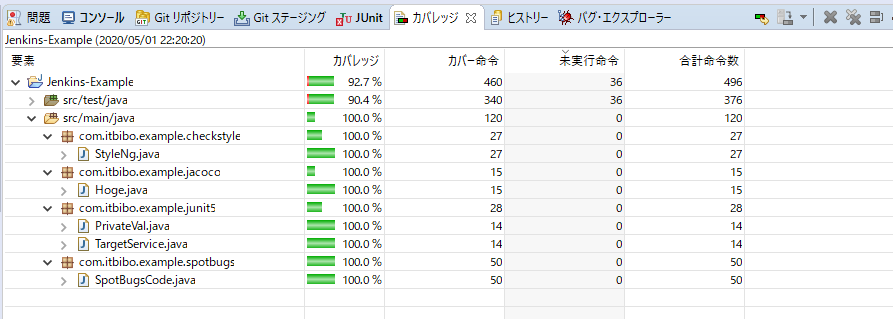
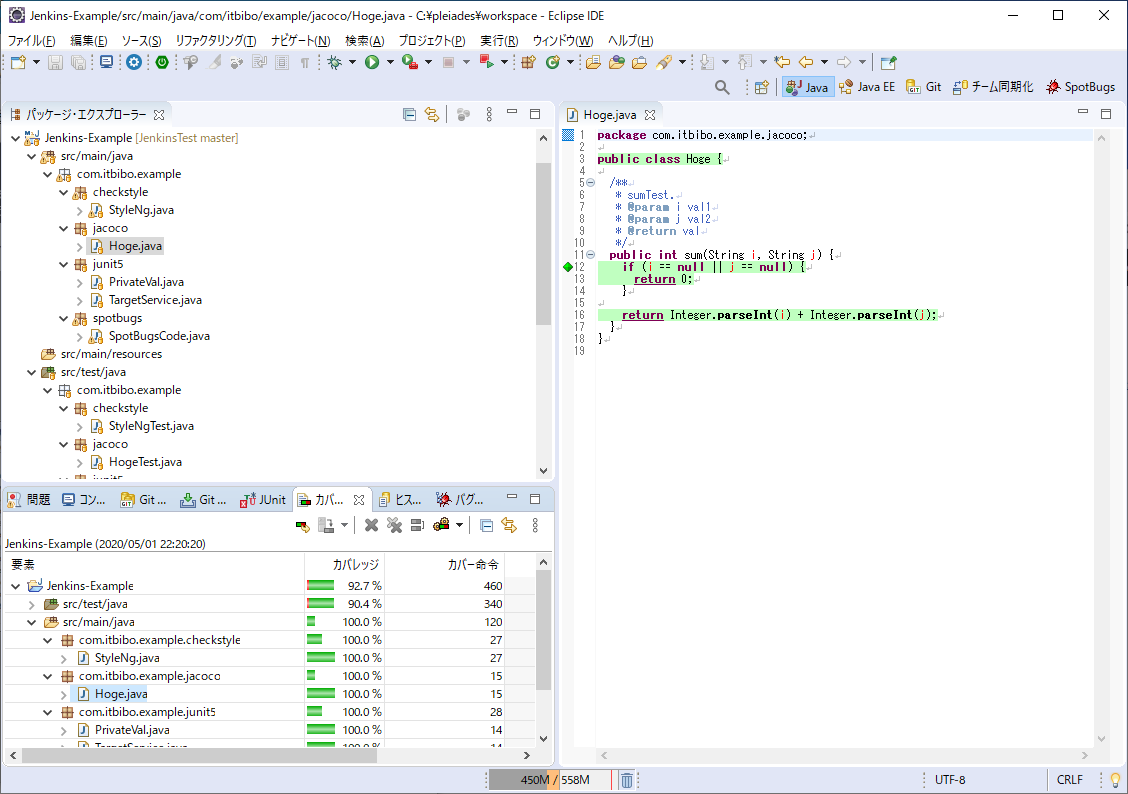
単体テスト(JUnit)
JUnitの実行手順です。
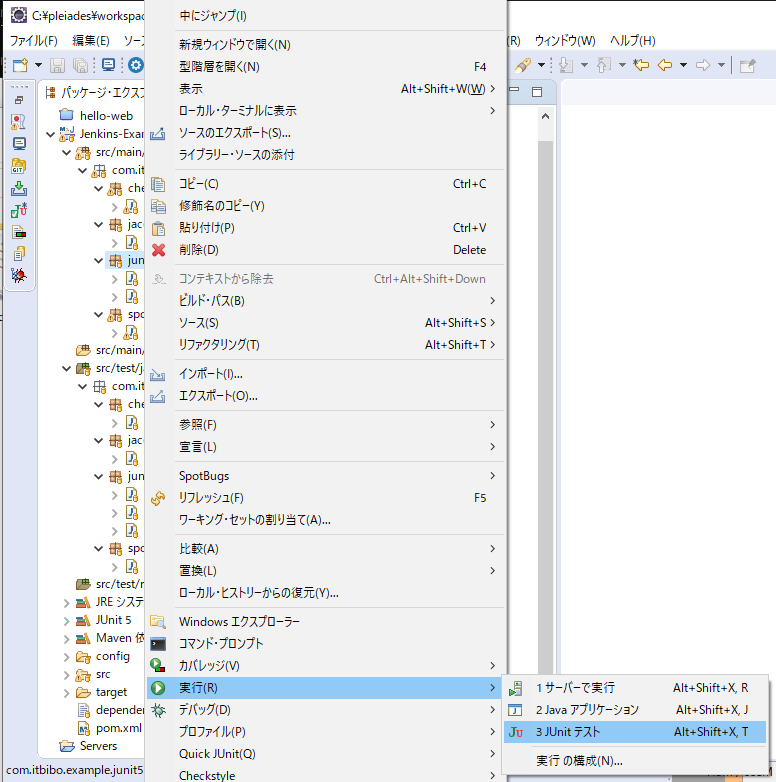
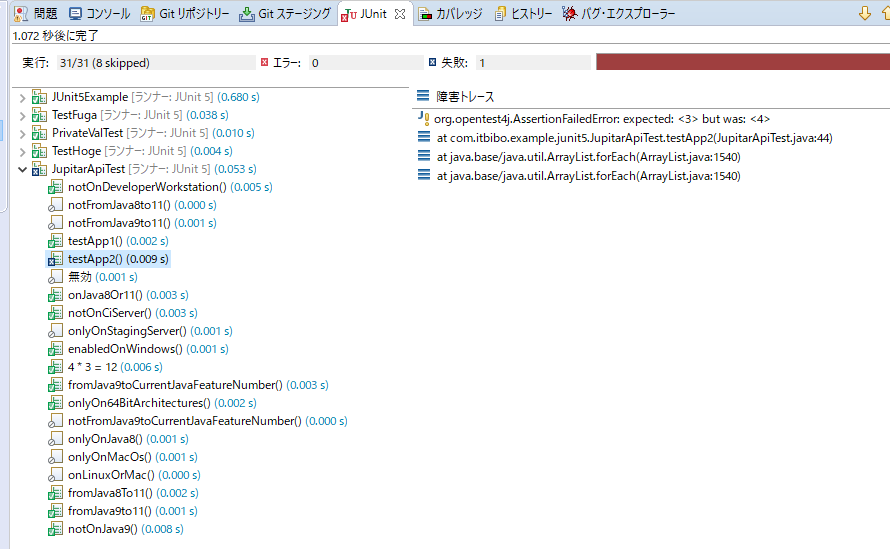
2+2=3 ではないですね。確認のため、わざとエラーを発生させています。
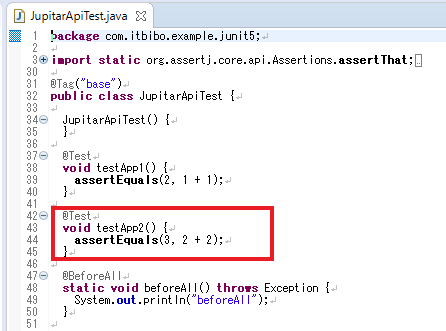
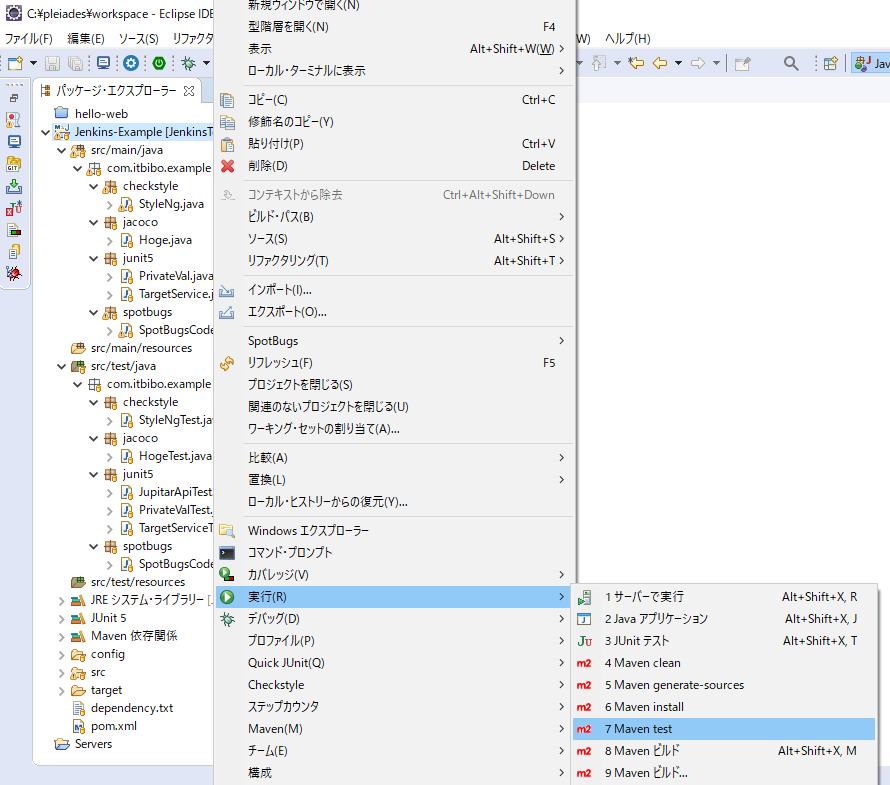
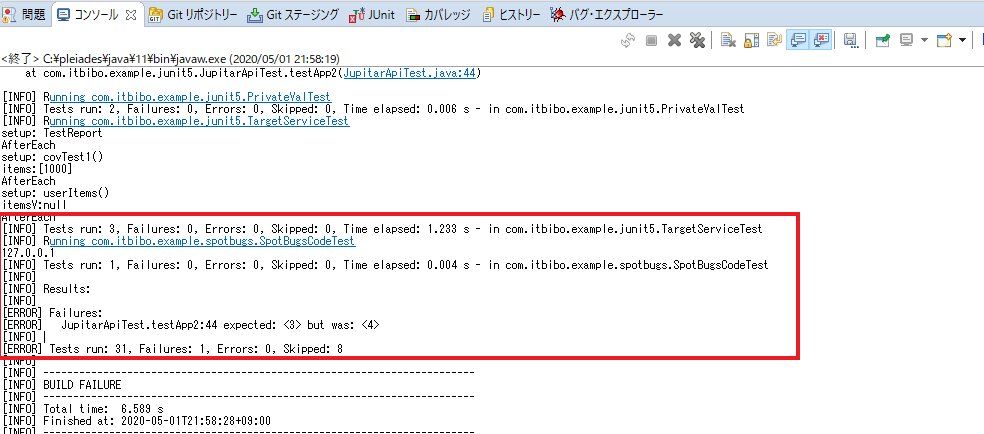
Jenkins連携確認
Jenkinsジョブの設定については、こちらの記事を参照してください。